curl 명령어나 postman 같은 프로그램을 사용하여도 되지만
VSCode에서 rest client 확장프로그램을 설치하여 api 호출을 해보자
설치
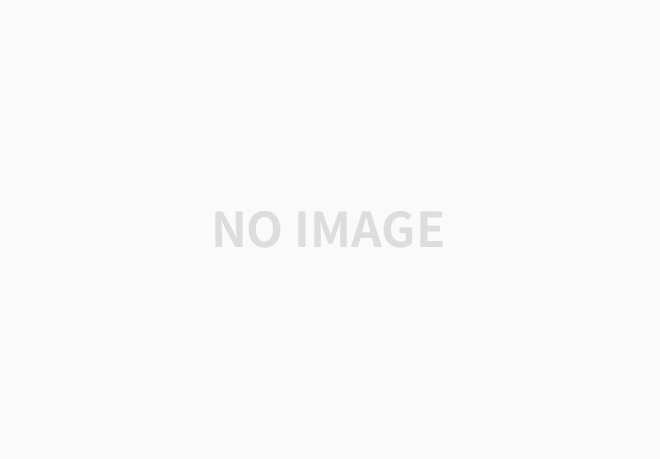
mongoose를 이용한 crud 코드
const express = require("express");
const bodyParser = require("body-parser");
const mongoose = require("mongoose");
const Person = require("./person-model");
mongoose.set("strictQuery", false);
const app = express();
app.use(bodyParser.json());
app.listen(3000, () => {
console.log("Server started");
const mongodbUri = "본인의 몽고DB 주소";
mongoose
.connect(mongodbUri, { useNewUrlParser: true })
.then(console.log("몽고DB 연결!"));
});
app.get("/person", async (req, res) => {
const person = await Person.find({});
res.send(person);
});
app.get("/person/:email", async (req, res) => {
const person = await Person.findOne({ email: req.params.email });
res.send(person);
});
app.post("/person", async (req, res) => {
const person = new Person(req.body);
await person.save();
res.send(person);
});
app.put("/person/:email", async (req, res) => {
const person = await Person.findOneAndUpdate(
{ email: req.params.email },
{ $set: req.body },
{ new: true }
);
console.log(person);
res.send(person);
});
app.delete("/person/:email", async (req, res) => {
await Person.deleteMany({ email: req.params.email });
res.send({ success: true });
});
http 파일 생성
@server = http://localhost:3000
### 전체 조회
GET {{server}}/person
### 생성
POST {{server}}/person
Content-Type: application/json
{
"name": "hjkang",
"age": 30,
"email": "test@test.com"
}
### 조회
GET {{server}}/person/test@test.com
### 수정
PUT {{server}}/person/test@test.com
Content-Type: application/json
{
"age": 29
}
### 삭제
DELETE {{server}}/person/test@test.com
그럼 이제 요청마다 "Send Request" 버튼이 생긴다.
해당 버튼을 클릭하여 요청할 수 있다
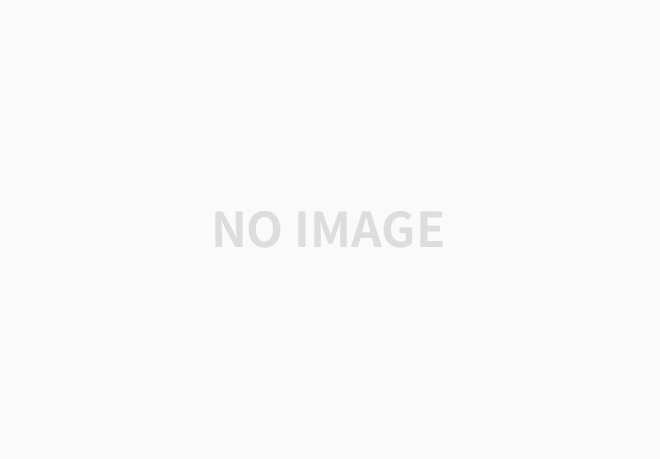
출력
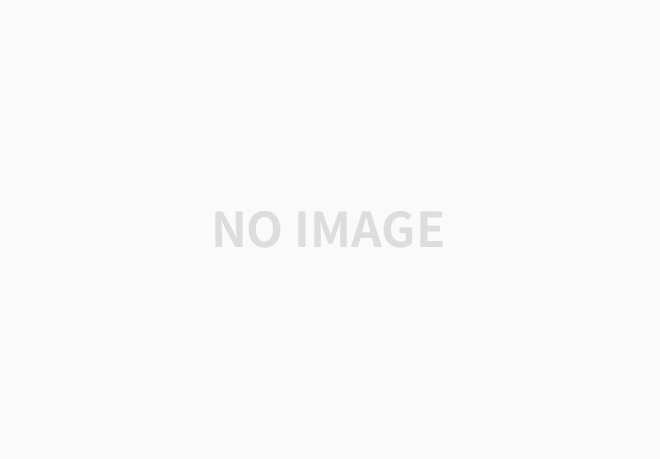
'ETC' 카테고리의 다른 글
HTTP 상태 코드 (0) | 2023.11.04 |
---|---|
OAuth란? (0) | 2023.10.01 |
모노레포(Monorepo) (0) | 2023.07.12 |
CSP, MSP 차이 (0) | 2023.05.08 |
Excel 그룹화 (부분합) (0) | 2023.02.15 |